This is a post by special contributor Andreas Loew, the creator of TexturePacker and PhysicsEditor.

Create this vertical scrolling platformer with Cocos2D!
Welcome back to the Monkey Jump tutorial! In this series, we are creating a fun vertical scrolling platformer with Cocos2D, TexturePacker and PhysicsEditor.
In Part One on the tutorial series, we introduced the MonkeyJump! game design, created the sprite sheets and shapes we needed, and began coding the game.
Before we stopped for a break, we had all of our game layers set up and had just finished making random objects drop from the sky, with sound effects.
In this second part of the tutorial, we will add our hero to the game, make him move and jump, and start adding some gameplay.
We’ll be starting with the project where we left off last time. If you don’t have it already, grab the source code for this tutorial series and open up 3-DraggingObjects.
Without further ado, let’s get back to (monkey) business! :]
Getting Started
We created the monkey’s shape in PhysicsEditor in Part One of this tutorial, but haven’t added the monkey to the game yet. Let’s do that now!
Add the Monkey class by creating a new file with the iOS\Cocoa Touch\Objective-C class template. Name the class Monkey, and make it a subclass of GB2Sprite. (Again, remember to change extension for the Monkey.m file to .mm)
The monkey will react to different events in the game world: he’ll put up his hands when something drops from above, push items, jump, etc. This is why you won’t be using Cocos2d’s standard animation routines, and instead implement some of your own.
For this, you need some member variables to store the additional data. Paste this code into Monkey.h replacing what’s there already:
#pragma once #import "Cocos2d.h" #import "GB2Sprite.h" @class GameLayer; @interface Monkey : GB2Sprite { float direction; // keeps monkey's direction (from accelerometer) int animPhase; // the current animation phase ccTime animDelay; // delay until the next animation phase is stated GameLayer *gameLayer; // weak reference } -(id) initWithGameLayer:(GameLayer*)gl; -(void) walk:(float)direction; @end |
Now switch to Monkey.mm and replace it with the following lines:
#import "Monkey.h" #import "GB2Contact.h" #import "GMath.h" #import "Object.h" #import "SimpleAudioEngine.h" #import "GameLayer.h" #define JUMP_IMPULSE 6.0f #define WALK_FACTOR 3.0f #define MAX_WALK_IMPULSE 0.2f #define ANIM_SPEED 0.3f #define MAX_VX 2.0f @implementation Monkey -(id) initWithGameLayer:(GameLayer*)gl { // 1 - Initialize the monkey self = [super initWithDynamicBody:@"monkey" spriteFrameName:@"monkey/idle/1.png"]; if(self) { // 2 - Do not let the monkey rotate [self setFixedRotation:true]; // 3 - The monkey uses continuous collision detection // to avoid getting stuck inside fast-falling objects [self setBullet:YES]; // 4 - Store the game layer gameLayer = gl; } return self; } @end |
Let’s go through the initWithGameLayer method step-by-step:
- First, we initialize the monkey. The monkey’s movement will be affected by the physics engine, so make him a dynamic object. We’ll use the idle frame as both the first frame of the monkey animation and the monkey’s physics shape.
- The monkey should stand straight up all the time, so we set his rotation to fixed. This means the monkey is moved by Box2d, but does not rotate or tilt.
- Set the monkey to bullet mode. Bullet mode enables continuous collision detection on an object. Without it, Box2d moves objects and then performs the collision checks. With fast-moving objects it’s possible that an object will pass through another without any collision detection at all or that an object will get stuck in another. Continuous collision detection calculates collisions all the way from an object’s current position to its new position – not just for the end point.
- Finally, you need to store the game layer – keep it as a weak reference and just assign the value.

A monkey bullet! Bullet mode enables continuous collision detection.
With regards to bullet mode for step #3, if you were coding a project with only a few objects, you could set the Box2d engine to run continuous collision detection for all game objects. However, when a game has a lot of objects that would add a lot of CPU overhead. So, for our game we’ll set the continuous mode on just the monkey and fast-moving (dropping) objects.
To enable the monkey, add him to the GameLayer. Open GameLayer.h and add the following lines at the top, just below the import statements:
@class Monkey; |
Now, add the following member variable to the GameLayer class:
Monkey *monkey; |
Then switch to GameLayer.mm and import Monkey.h at the top of the file:
#import "Monkey.h" |
At the end of the init selector in GameLayer.mm, initialize the monkey, add him to the game layer and set a starting position for the monkey:
monkey = [[[Monkey alloc] initWithGameLayer:self] autorelease]; [objectLayer addChild:[monkey ccNode] z:10000]; [monkey setPhysicsPosition:b2Vec2FromCC(240,150)]; |
Compile and run, and you’ll see the following:

The monkey is in the house! Objects drop onto him and he gets pushed away – perfect. That’s exactly what we want.
Do the Monkey Walk
Our next goal is to make the monkey walk, using the accelerometer as input.
Go back to GameLayer.mm and add the following code at the end of the init method:
self.isAccelerometerEnabled = YES; |
This will ensure that for each change in the built-in accelerometer values, the GameLayer class gets an automatic notification. The notification handler has to be added to GameLayer.mm at the end of the file, before the @end marker:
- (void)accelerometer:(UIAccelerometer*)accelerometer didAccelerate:(UIAcceleration*)acceleration { // forward accelerometer value to monkey [monkey walk:acceleration.y]; } |
The accelerometer handler calls the walk method of the monkey object with the y-axis value of the accelerometer. This method will handle actually moving the monkey back and forth based on the accelerometer input.
So, move to Monkey.mm and add the walk method to the end of the file (before the @end marker). This method simply stores the new movement direction for the monkey in a member variable.
-(void) walk:(float)newDirection { direction = newDirection; } |
Try compiling and running the code now … Surprise! Nothing new happens. This is due to the fact that while the direction value has been stored, it has not been applied to the physics simulation yet. In order to update the physics simulation based on the new movement direction, we need to override the updateCCFromPhysics selector, which is called by the GB2Engine on every frame for a GB2Node object to update the physics.
Update Monkey Physics
Add the following code to Monkey.mm:
-(void) updateCCFromPhysics { // 1- Call the super class [super updateCCFromPhysics]; // 2 - Apply the directional impulse float impulse = clamp(-[self mass]*direction*WALK_FACTOR, -MAX_WALK_IMPULSE, MAX_WALK_IMPULSE); [self applyLinearImpulse:-b2Vec2(impulse,0) point:[self worldCenter]]; } |
In the above, you first call the selector of the super class. This will update the monkey’s sprite based on physics simulation.
Then, you push the monkey in the right direction based on the stored direction value. It is important not to take complete control of the monkey. Otherwise, his “natural” behavior in response to events such as items dropping or collision detection won’t work properly.
All you really do is to give the monkey a nudge in the right direction. Since the physics engine updates happen 60 times per second, it’s important to keep the push quite light. It’s a monkey – not a bullet, even if he is in bullet mode!
You can move a box2D object by applying an impulse to the object. And you do this using the GB2Sprite’s applyLinearImpulse method, which takes two parameters: the impulse to apply and the point of application.
For the point of application, we’ll use the world center of the object. Applied at the world center, the object will be pushed without any torque that would result into a rotation. (Which, by the way, would not happen to the monkey anyway, since we already set him not to rotate.)

When applying an impulse, I recommend using the mass of the object, which you can get with [self mass]. This is because the impulse is the product of mass and velocity.
Multiply the mass value by the stored direction value. This gives you small impulses when the device is tilted slightly, and bigger impulses when its tilted sharply.
Scaling the impulse with the object’s mass frees us from having to worry about the movement changing when we change the object’s shape in PhysicsEditor. If we didn’t scale the impulse and later we made the monkey a bit smaller (for example), the same impulse applied to a monkey of less mass will result in a faster-moving monkey.
We will also clamp the value to a maximum to avoid impulses that are too strong. The maximum impulse is defined with the MAX_WALK_IMPULSE variable.
Compile and run. Still nothing? Ah, I forgot to tell you one thing: the iPhone simulator does not simulate the accelerometer. So, from now on, we need to test on the device! Switch to the device and test.
The monkey now slides left and right – but the movement doesn’t look very natural.
Make the Monkey Move
We’re going to add some code to Monkey.mm to get the monkey animations working. Add the following to the end of the updateCCFromPhysics method:
animDelay -= 1.0f/60.0f; if(animDelay <= 0) { animDelay = ANIM_SPEED; animPhase++; if(animPhase > 2) { animPhase = 1; } } |
The first line simply updates the time till the next animation by decreasing the time delay till the next animation phase. I use the value 1.0f/60.0f because I assume that the application runs at 60 fps and the updateCCFromPhysics method does not have a delta time parameter which would provide the timer interval between each update accurately.
If the animation time delay drops below zero, reset the animation delay value to the animation speed and increase the current phase by one. If the highest phase is reached, loop back to 1 so that the animation will continue to play in a loop.
Next, we need to determine the direction the monkey is facing. There are two ways to do this:
- Use the direction from the accelerometer
- Use the monkey’s velocity vector
I prefer to use the accelerometer, since it gives the player immediate feedback when he or she tries to change the direction by tilting the device. We’ll respond to velocity changes via accelerometer later.
Add this code to the end of updateCCFromPhysics:
// determine direction of the monkey bool isLeft = (direction < 0); // direction as string NSString *dir = isLeft ? @"left" : @"right"; // update animation phase NSString *frameName; const float standingLimit = 0.1; float vX = [self linearVelocity].x; if((vX > -standingLimit) && (vX < standingLimit)) { // standing frameName = [NSString stringWithFormat:@"monkey/idle/2.png"]; } else { // walking NSString *action = @"walk"; frameName = [NSString stringWithFormat:@"monkey/%@/%@_%d.png", action, dir, animPhase]; } // set the display frame [self setDisplayFrameNamed:frameName]; |
Basically, all the code above does is, if the monkey’s speed is lower than standingLimit, it makes him look directly at the player with his idle animation frame. Otherwise, it uses a walk display frame matching the current direction and animation frame number.
Compile and run. The monkey now runs about – nice!
Slow Down Little Fella
I’m still not happy with one thing: I think the monkey moves too fast. We could reduce the impulse we’re applying to make him walk, but this will also make him slow and clumsy.
We need a strong enough impulse to make him react fast – but not too fast.
Replace the current code for updateCCFromPhysics in Monkey.mm with the following code:
// 1 - Call the super class [super updateCCFromPhysics]; // 2 - Update animation phase animDelay -= 1.0f/60.0f; if(animDelay <= 0) { animDelay = ANIM_SPEED; animPhase++; if(animPhase > 2) { animPhase = 1; } } // 3 - Get the current velocity b2Vec2 velocity = [self linearVelocity]; float vX = velocity.x; // 4 - Determine direction of the monkey bool isLeft = (direction < 0); if((isLeft && (vX > -MAX_VX)) || ((!isLeft && (vX < MAX_VX)))) { // apply the directional impulse float impulse = clamp(-[self mass]*direction*WALK_FACTOR, -MAX_WALK_IMPULSE, MAX_WALK_IMPULSE); [self applyLinearImpulse:-b2Vec2(impulse,0) point:[self worldCenter]]; } // 5 - Get direction as string NSString *dir = isLeft ? @"left" : @"right"; // 6 - Update animation phase NSString *frameName; const float standingLimit = 0.1; if((vX > -standingLimit) && (vX < standingLimit)) { // standing frameName = [NSString stringWithFormat:@"monkey/idle/2.png"]; } else { // walking NSString *action = @"walk"; frameName = [NSString stringWithFormat:@"monkey/%@/%@_%d.png", action, dir, animPhase]; } // 7 - Set the display frame [self setDisplayFrameNamed:frameName]; |
As you’ll notice, we’ve moved a few code blocks around but the major change is the addtion of a new line to section #3 for the vX variable and moving the impulse code to section #4 to be wrapped within an if condition that checks if the velocity is below a maximum value for the current direction. This allows the monkey to steer against being pushed away by an object, but keeps him from accelerating too fast on his own.
Compile and run. I thinks this looks much better now.

The source code of the project in its current state is available in the folder 4-WalkingMonkey.
Jump, Jump!
Now, let’s make the monkey jump. For this, we need to make the game detect touch events anywhere on the GameLayer, because we want the monkey to jump at every touch.
Open GameLayer.mm and enable touch detection by adding the following line to the init selector:
// enable touches self.isTouchEnabled = YES; |
Also add the following selector at the end of the file (but before the @end marker). It forwards the touches to the monkey object via the jump method.
-(void)ccTouchesBegan:(NSSet *)touches withEvent:(UIEvent *)event { [monkey jump]; } |
You might notice at this point that we don’t have a jump method in the Monkey class. That’s what we’ll add next. Switch to Monkey.h and add the following method definition just before @end:
-(void) jump; |
Now open Monkey.mm and add the following code before the @end marker:
-(void) jump { [self applyLinearImpulse:b2Vec2(0,[self mass]*JUMP_IMPULSE) point:[self worldCenter]]; [[SimpleAudioEngine sharedEngine] playEffect:@"jump.caf" pitch:gFloatRand(0.8,1.2) pan:(self.ccNode.position.x-240.0f) / 240.0f gain:1.0 ]; } |
All the above code does is to apply an impulse to make the monkey jump and play the jump sound. We’ll pan the sound as we did for the object sounds in Part One of this tutorial.
Compile and run. Tap the screen to make the monkey jump.
Outta Sight!
But this is far from perfect: if you tap the screen multiple times, the monkey goes through the roof! Worse still, the camera does not follow the monkey.
Let’s fix the camera first. First, add the following code to the top of the update selector, above the existing code, in GameLayer.mm.
// 0 - monkey's position float mY = [monkey physicsPosition].y * PTM_RATIO; |
The above code copies the monkey’s y-position into a new variable mY. Of course, we could also access the monkey’s ccNode and take the y-coordinate from there. The end result will be the same as multiplying his physics position by the PTM_RATIO.
Now, add these lines to the end of the update selector after the closing curly brace after section #7.
// 8 - Adjust camera const float monkeyHeight = 70.0f; const float screenHeight = 320.0f; float cY = mY - monkeyHeight - screenHeight/2.0f; if(cY < 0) { cY = 0; } |
Here, we calculate a good value for the camera’s y-coordinate, one that will more or less center the monkey on the middle of the screen. Clamp the value so that it does not go below 0 so that the camera does not move below ground level.
Now let’s implement parallax scrolling of the background so that the monkey’s movement appears more natural. The effect is quite easy to accomplish: just multiply the background layer by a factor below 1.0 and set the position for the layers. This will make the background layer scroll slower. The further away a layer is from the camera, the smaller the factor must be.
Add this code below the last few lines added to update:
// 9 - Do some parallax scrolling [objectLayer setPosition:ccp(0,-cY)]; [floorBackground setPosition:ccp(0,-cY*0.8)]; // move floor background slower [background setPosition:ccp(0,-cY*0.6)]; // move main background even slower |
Feel free to adjust the values to your liking.
That’s it – compile and run. Tap the screen multiple times to see the monkey rise. And beware of flying monkeys!!!!

And It All Piles Up!
If you play the game for a while, you’ll notice that the items continue to drop from the same fixed height – sometimes way below the monkey’s current position. In fact, after some time, items don’t even drop because they’ve piled up beyond the spawning point.
Fix this by changing the following line in GameLayer.mm‘s update method:
float yPos = 400; |
Change the above line to this:
float yPos = 400 + mY; |
Items will now spawn 400pt above the monkey’s head, wherever it may be.
Back to the monkey. You’ll have noticed that the monkey can actually jump again and again while up in the air. This just won’t do. We need to fix it so the monkey only jumps when he has contact with the floor.
Let’s start by counting the number of contacts the monkey makes with the floor.
Add a new variable to Monkey.h:
int numFloorContacts; // number of floor contacts |
Switch to Monkey.mm and add the following two new collision detection handlers to the end of the file (but above the @end marker):
-(void) beginContactWithFloor:(GB2Contact*)contact { numFloorContacts++; } -(void) endContactWithFloor:(GB2Contact*)contact { numFloorContacts--; } |
As the names imply, the first one detects the beginning of a contact/collision with the floor and the second the end of a collision. In the beginContact selector, we increase the value of the floor contact variable, and then decrease it in the endContact selector.
These selectors are going to get called by GBox2D every time a contact starts or ends between the monkey and the floor. (Remember: we created a separate class for the floor so that GBox2D now can call the appropriate selector with the class’s name).
Now, if the monkey is standing on the floor, then the numFloorContacts value should be at least one. Use this to our advantages by wrapping the code in the jump method in Monkey.mm with an if condition to see if the monkey is actually standing on the floor before jumping:
-(void) jump { if(numFloorContacts > 0) { [self applyLinearImpulse:b2Vec2(0,[self mass]*JUMP_IMPULSE) point:[self worldCenter]]; ... } } |
Compile and run. Everything seems fine. Well… except that when the monkey lands on an object, he loses the ability to jump. To fix this, we’re going to consider any contact the monkey has with objects similar to a contact with the floor.
And it’s very simple to implement. Simply add a couple more collision-handling routines to the end of Monkey.mm and count object contacts the same way we counted floor contacts:
-(void) beginContactWithObject:(GB2Contact*)contact { // count object contacts as floor contacts numFloorContacts++; } -(void) endContactWithObject:(GB2Contact*)contact { // count object contacts as floor contacts numFloorContacts--; } |
Compile and run. Isn’t it much better now? And our game is already playable!
Push It!
Let’s improve the gameplay and allow the monkey to push objects to the left and right – and give him the ability to put his hands above his head to shield him from dropping objects.
Do you remember how you added the sensors to the left and right sides of the monkey in Part One? They come into play now! The key to the sensors was setting up the “Id” parameter in PhysicsEditor. You are going to retrieve this value now!
But before we do that, we need to add a few instance variables to keep count of the left and right sensors, as well as the number of contacts made with the monkey’s head. Add these variables to Monkey.h:
int numPushLeftContacts; int numPushRightContacts; int numHeadContacts; |
Next, the beginContactWith* and endContactWith* selectors have a contact parameter we can use to determine which part of the monkey has contact with an object – the “Id” value we added in PhysicsEditor is stored as user data in each fixture. So replace the existing object contact handlers for Monkey.mm with the following:
-(void) beginContactWithObject:(GB2Contact*)contact { NSString *fixtureId = (NSString *)contact.ownFixture->GetUserData(); if([fixtureId isEqualToString:@"push_left"]) { numPushLeftContacts++; } else if([fixtureId isEqualToString:@"push_right"]) { numPushRightContacts++; } else if([fixtureId isEqualToString:@"head"]) { numHeadContacts++; } else { // count others as floor contacts numFloorContacts++; } } -(void) endContactWithObject:(GB2Contact*)contact { NSString *fixtureId = (NSString *)contact.ownFixture->GetUserData(); if([fixtureId isEqualToString:@"push_left"]) { numPushLeftContacts--; } else if([fixtureId isEqualToString:@"push_right"]) { numPushRightContacts--; } else if([fixtureId isEqualToString:@"head"]) { numHeadContacts--; } else { // count others as floor contacts numFloorContacts--; } } |
As you see from the new code, you retrieve the Id, which we’ll call fixtureId here, by accessing the contact parameter’s fixture and then accessing the fixture’s user data via the GetUserData method.
Now that we’re tracking the contacts, we can update the monkey’s animation frames to handle additional events.
Here’s the decision table for the various animations:
Standing | No object above head | Idle |
Standing | Object above head | Arms up |
Moving | No push sensor active in the direction | Walk |
Moving | Push sensor active in the direction | Push |
Using the above table, we modify section #6 of updateCCFromPhysics in Monkey.mm as follows:
// 6 - Update animation phase const float standingLimit = 0.1; NSString *frameName = nil; if((vX > -standingLimit) && (vX < standingLimit)) { if(numHeadContacts > 0) { // Standing, object above head frameName = [NSString stringWithFormat:@"monkey/arms_up.png"]; } else { // Just standing frameName = [NSString stringWithFormat:@"monkey/idle/2.png"]; } } else { if(numFloorContacts == 0) { // Jumping, in air frameName = [NSString stringWithFormat:@"monkey/jump/%@.png", dir]; } else { // Determine if monkey is pushing an item bool isPushing = (isLeft && (numPushLeftContacts > 0)) || (!isLeft && (numPushRightContacts > 0)); // On the floor NSString *action = isPushing ? @"push" : @"walk"; frameName = [NSString stringWithFormat:@"monkey/%@/%@_%d.png", action, dir, animPhase]; } } |
Compile and test. Perfect! The monkey now behaves just as we want.

It Takes a Strong Monkey …
Playing a little bit more though, I think the monkey should be a bit stronger under certain conditions. Currently, he’s too weak to break free when an object is above his head. Let’s give him some extra strength when he’s trapped like that and wants to jump.
This is the current line from the jump selector in Monkey.mm that makes the monkey jump:
[self applyLinearImpulse:b2Vec2(0,[self mass]*JUMP_IMPULSE) point:[self worldCenter]]; |
Replace it with:
float impulseFactor = 1.0; // if there is something above monkey's head make the push stronger if(numHeadContacts > 0) { impulseFactor = 2.5; } [self applyLinearImpulse:b2Vec2(0,[self mass]*JUMP_IMPULSE*impulseFactor) point:[self worldCenter]]; |
That’s our monkey on steroids! He now uses a 2.5-times stronger impulse when there’s an object resting above him – this should allow him to break free of most of the objects.
Let’s also change the walking impulse in case the monkey needs to push an object to the side to break free. Go to updateCCFromPhysics and cut the following lines from section #6:
// Determine if monkey is pushing an item bool isPushing = (isLeft && (numPushLeftContacts > 0)) || (!isLeft && (numPushRightContacts > 0)); |
Now paste that code into section #4 and modify it as follows:
// 4 - Determine direction of the monkey bool isLeft = (direction < 0); // Determine if monkey is pushing an item bool isPushing = (isLeft && (numPushLeftContacts > 0)) || (!isLeft && (numPushRightContacts > 0)); if((isLeft && (vX > -MAX_VX)) || ((!isLeft && (vX < MAX_VX)))) { // apply the directional impulse float impulse = clamp(-[self mass]*direction*WALK_FACTOR, -MAX_WALK_IMPULSE, MAX_WALK_IMPULSE); if(isPushing) { impulse *= 2.5; } [self applyLinearImpulse:-b2Vec2(impulse,0) point:[self worldCenter]]; } |
Compile and test – that’s much better. But there’s still a problem: when the monkey slightly grazes an object with his head, the new jump power makes him go through the roof!
We need to clamp his maximum speed inside the updateCCFromPhysics method. Add this to the end of section #3 of the updateCCFromPhysics method:
const float maxVelocity = 5.0; float v = velocity.Length(); if(v > maxVelocity) { [self setLinearVelocity:maxVelocity/v*velocity]; } |
Notice that in the code above we are directly modifying values controlled by the Box2d engine, thus affecting the overall behaviour of the physics engine. You should try to avoid doing this kind of manipulation.
Compile and test. I like the monkey’s behavior now. He reacts quickly, and is strong enough to push objects but does not become uncontrollable.
Where To Go From Here?
If you don’t have it already, here is all of the source code for this tutorial series.
You’ve now reached the end of Part Two of the MonkeyJump tutorial! The project in its current form is available in the source code zip in the folder called 5-MonkeyJumpAndRun.
Stay tuned for the final part of the series, where we’ll add some performance improvements, add a HUD layer to the game, and yes – kill the monkey! :]
In the meantime, if you have any questions or comments, please join the forum discussion below!
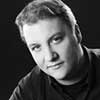
How To Build a Monkey Jump Game Using Cocos2D, PhysicsEditor & TexturePacker Part 2 is a post from: Ray Wenderlich
没有评论:
发表评论